
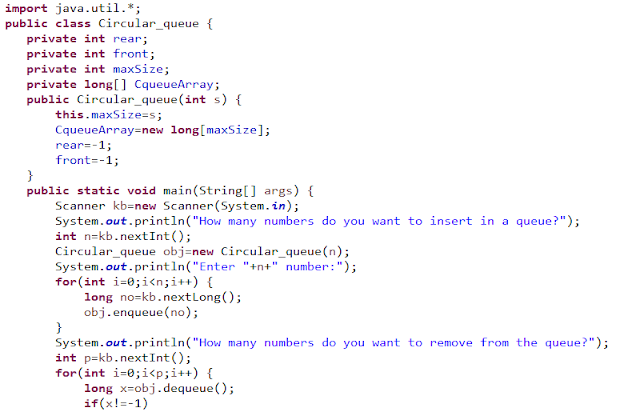
# Circular Queue implementation in Python for the last element, reset the values of FRONT and REAR to -1.circularly increase the FRONT index by 1.add the new element in the position pointed to by REAR.if the rear reaches the end, next it would be at the start of the queue) circularly increase the REAR index by 1 (i.e.for the first element, set value of FRONT to 0.initially, set value of FRONT and REAR to -1.REAR track the last elements of the queue.FRONT track the first element of the queue.If REAR + 1 = 5 (overflow!), REAR = (REAR + 1)%5 = 0 (start of queue) Here, the circular increment is performed by modulo division with the queue size. when we try to increment the pointer and we reach the end of the queue, we start from the beginning of the queue. This reduces the actual size of the queue.Ĭircular Queue works by the process of circular increment i.e. Here, indexes 0 and 1 can only be used after resetting the queue (deletion of all elements). In a normal queue, after a bit of insertion and deletion, there will be non-usable empty space. The circular queue solves the major limitation of the normal queue. Decrease Key and Delete Node Operations on a Fibonacci HeapĪ circular queue is the extended version of a regular queue where the last element is connected to the first element.PriorityQueue numbers = new PriorityQueue(new CustomComparator()) However, we can customize this ordering.įor this, we need to create our own comparator class that implements the Comparator interface. In all the examples above, priority queue elements are retrieved in the natural order (ascending order). Returns the length of the priority queue.Ĭonverts a priority queue to an array and returns it. If the element is found, it returns true, if not it returns false. Searches the priority queue for the specified element. Output PriorityQueue using iterator(): 1, 4, 2, In order to use this method, we must import the package. To iterate over the elements of a priority queue, we can use the iterator() method. ("Removed Element Using poll(): " + number) poll() - returns and removes the head of the queue.remove() - removes the specified element from the queue.This method returns the head of the queue. To access elements from a priority queue, we can use the peek() method. The queue is now rearranged to store the smallest element 1 to the head of the queue. It is because the head of the priority queue is the smallest element of the queue. We have inserted 4 and 2 to the queue.Īlthough 4 is inserted before 2, the head of the queue is 2. Here, we have created a priority queue named numbers. PriorityQueue numbers = new PriorityQueue() If the queue is full, it returns false.įor example, import offer() - Inserts the specified element to the queue.If the queue is full, it throws an exception. add() - Inserts the specified element to the queue.The PriorityQueue class provides the implementation of all the methods present in the Queue interface. We will learn about that later in this tutorial. However, we can customize the ordering of elements with the help of the Comparator interface. And elements are removed in ascending order from the queue. In this case, the head of the priority queue is the smallest element of the queue.
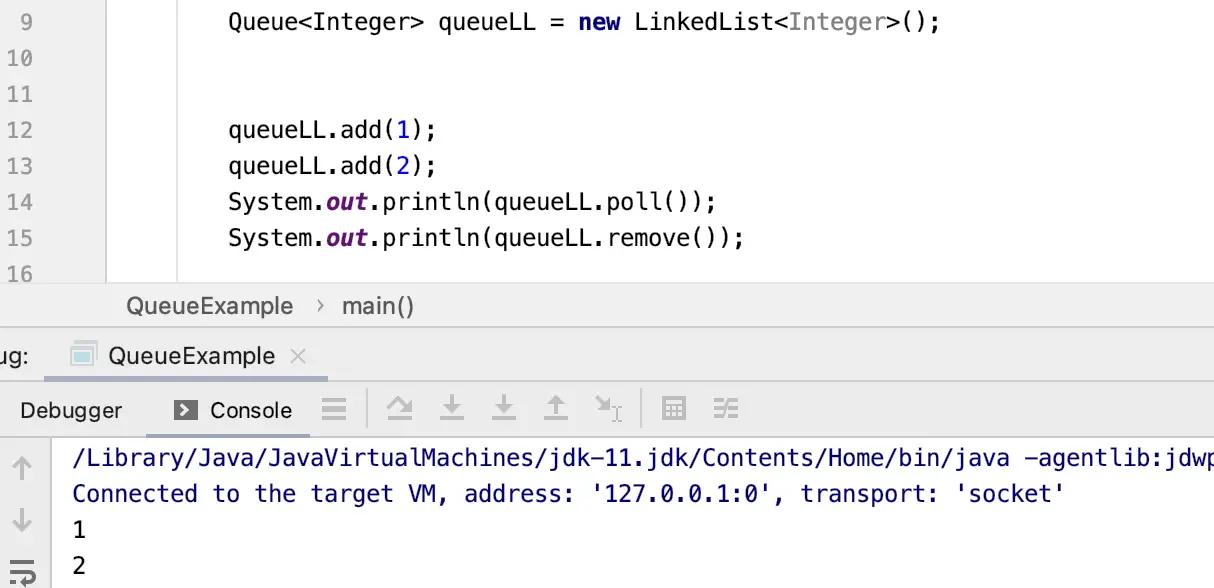
Here, we have created a priority queue without any arguments. Once we import the package, here is how we can create a priority queue in Java. In order to create a priority queue, we must import the package.
However, elements are always retrieved in sorted order. It is important to note that the elements of a priority queue may not be sorted. Once this element is retrieved, the next smallest element will be the head of the queue. In this case, the head of the priority queue will be the smallest element. Suppose, we want to retrieve elements in the ascending order. Unlike normal queues, priority queue elements are retrieved in sorted order. The PriorityQueue class provides the functionality of the heap data structure.
